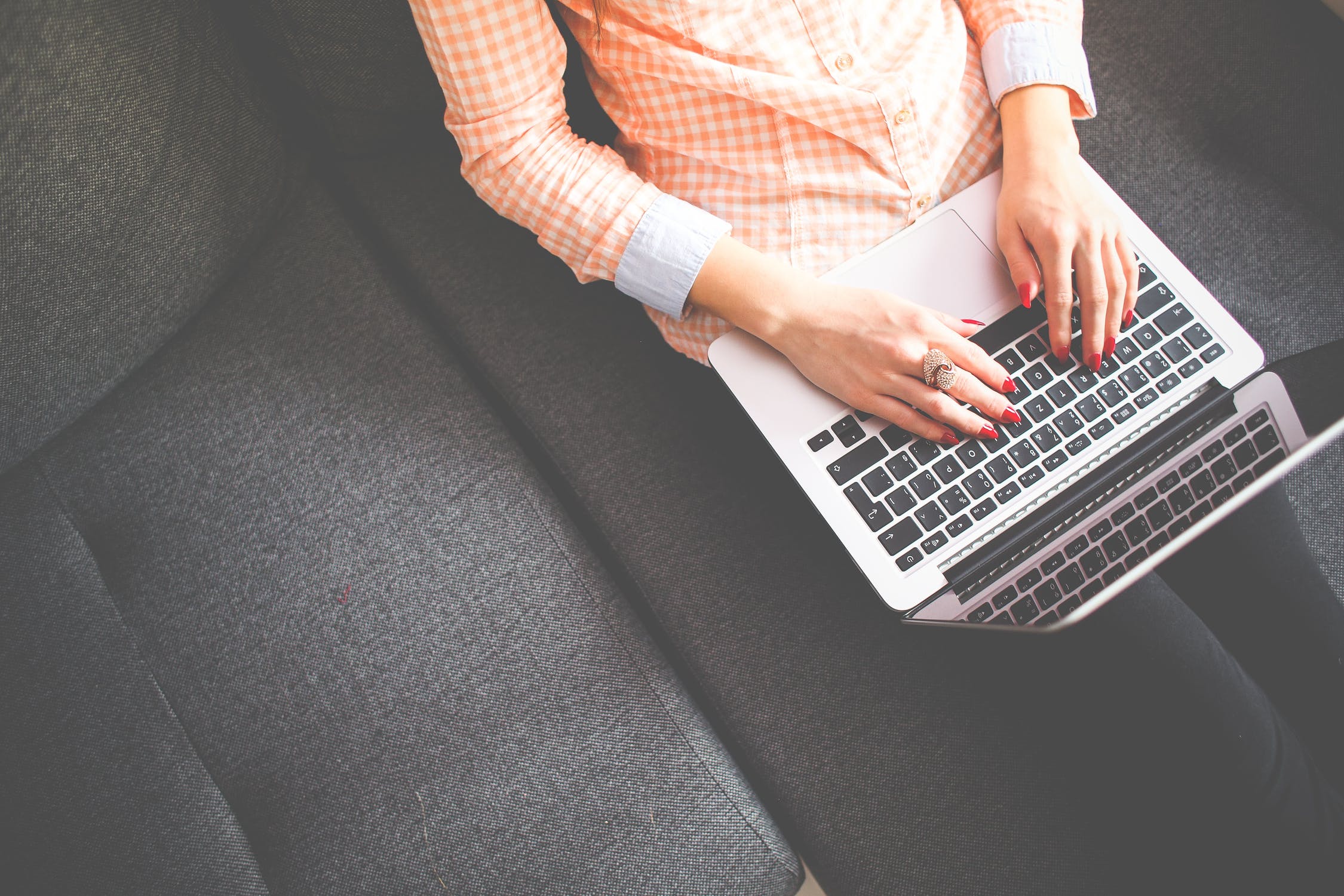
Setting Up A Basic Rich Text Editor in React
React is an open-source JavaScript library that allows you to establish user interfaces for one-page applications. With React, you can make front-end web application development in a hassle-free way. Meanwhile, you need a React rich text editor for the customization of your project. One of the rich text editors you can use is TinyMCE.
Before we proceed to the process of setting up your rich text editor in React, let us first know more about React.
Features of React
React comes with functional features that you can use to create your project successfully. The following are the features of React.
Virtual document object model
React allows you to make an in-memory data structure cache that can compute changes. Then, it will update your browser. It helps you to code easier.
Easy to learn
Another advantage of React is that, it is easy to learn. It doesn’t need knowledge in programming. With React, you just need basic HTML and CSS knowledge.
Easy to test
React is also easy to test. You can check the triggered actions, output, functions, events, and more.
If you want a flexible and innovative React WYSIWYG text editor, TinyMCE will not fail you. It is loaded with amazing features that can meet your specific needs. In this article, we will discuss setting up a basic rich text editor in React.
Rich Text Editor Setup in React
Use React form as a controlled component
The first thing to do is to make a React project through Create React App. Then, you can open src/ App.js file and change the code with the following:
import React from “react” ;
class App extends React.Component {
constructor (props) {
super(props) ;
this.state = { content: “”};
this.handleChange = this.handleChange. bind (this);
this.handleSubmit = this.handleSubmit. bind (this);
}
handleChange (event) {
this.setState ({ content: event.target.value });
}
handleSubmit (event) {
alert (“Text was submitted : “ + this.state.content);
event.preventDefault ();
}
render( ) {
return (
<form onSubmit = [this.handleSubmit} >
<h2>Introduction to Software Engineering </h2>
<h3>Provide a course overview</ h3>
<textarea
rows=”20”
cols = “80”
value = {this.state.content}
onChange = {this.handleChange}
/>
<br />
<input type = “submit” value= “Submit” />
</form>
);
}
}
export default App;
The next step is to test it. You can run the npm run start in the project directory. Upon the compilation, you can get a URL. You can open this URL in a browser, and you can get a simple React form as a controlled component.
Add rich text editor in the React form
You can now add your rich text editor in the React form. You can install the tinymce-react component on your chosen package manager. For example, if you use npm:
$ npm install @tinymce/tinymce-react
You can open the src/App.js file. Then, you can import the Editor component by adding it to the import list.
import React from “react” ;
import { Editor } from “@tinymce/tinymce-react” ;
In similar file, remove the textarea from the form, change it with Editor component:
Test it
<form onSubmit={this.handleSubmit}>
<h2>Introduction to Software Engineering</h2>
<h3>Provide a course overview</h3>
<Editor
value = {this.state.content}
init = {{
height: 500,
menubar: false
} }
onEditorChange = {this.handleChange}
/>
<br />
<input type = “submit” value = “Submit” />
</form>
The value property is designed to set the initial content. On the other hand, the init property is made to configure the editor. Fortunately, TinyMCE is flexible when it comes to configuration. You can use it for different applications.
The onEditorChange property can be used to handle event, it may need change on the props by the handleChange ( ) function:
handleChange(content, editor) {
This.setState( {content} );
}
You can save the changes you made. Then, you can have a rich text editor to your React form.
Register your domain with Tiny Cloud
The next step is to register with your React rich text editor, which is the TinyMCE.
- Avail of your free API key. You can also get a 30-day trial for their premium plugins.
- Add your domain to the Approved Domains in your Tiny account.
- Add the API key to the editor config in src/App.js.
Customize your editor
Once you get your default rich text editor with your React form, you can now customize it. TinyMCE comes with customization tools that can suit your specific needs.
Meanwhile, aside from TinyMCE, the following are other rich text editors you can use for React.
Other Rich Text Editors for React
Suneditor React
The Suneditor is flexible and lightweight for your web applications. It can be used as CodeMirror and allows you to paste from Excel and Microsoft Word.
React RTE
It is a full-featured textarea replacement like TinyMCE, CKEditor as well as other rich text WYSIWYG editors.
CKEditor React
CKEditor is an innovative JavaScript rich text editor that comes with modular architecture. With its features, you can make semantic content easily.
Draft.js
Draft.js is another framework you can use to build rich text editors in React. You can make rich text input with this editor.
React Prosemirror
React Prosemirror can be your efficient tool to establish rich text editors on the web. It is equipped with functional features for your project.
Conclusion
To sum it up, setting up your basic rich text editor in React is easy. You can follow the steps mentioned above for your front-end development process.